MkObj - VanillaJS Library for Instance-safe objects
- December 27, 2014
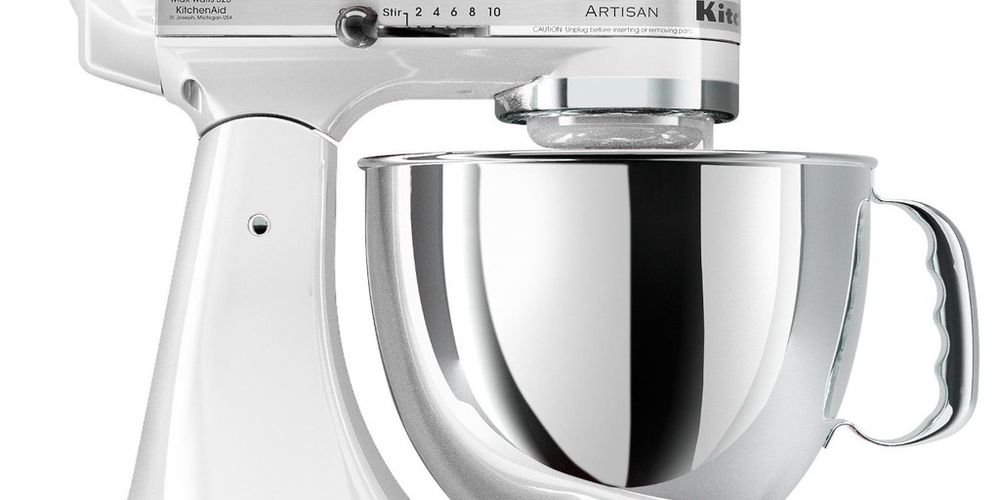
Part of my job as an instructor at Lighthouse Labs is to teach Prototypal Inheritance in JavaScript. It’s usually left for one of the advanced lectures in the later part of the bootcamp, and also typically turns students’ heads on one end and spins them around when they first wrap their heads around the idea that all inheritance isn’t like class-based inheritance in Ruby, for instance. (pardon the pun)
However, I recently came upon a very interesting article/talk by Eric Elliott entitled “The Two Pillars of Javascript”. In it, Elliott brings up the very important point that in JavaScript, new objects created through “typical” prototypal inheritance are not instance-safe. This means that when you change the prototype of the ‘parent’ class, you are also changing the implementation of the inheriting class/object/child.
As an example, see this code:
As you can see if you run this code, what ends up happening is that our Garfield instance gets the swish() method we add to Cat, even though it wasn’t part of the original inheritance.
So, I started working on an implementation of some of the principles that Elliott covered in his talk, without having to implement something the size and weight of StampIt(his library for building instance safe objects). It wasn’t that I thought StampIt was a bad idea. In fact, it’s great. But what intrigued me was the idea of mixin-based object creation, where you could throw a few methods and properties in a mortar and pestle, grind for thirty seconds, and out pops an object.
So, I have created MkObj JS Object Mixin Library, a simple implementation which allows you to register callbacks and properties which you would like to use in object construction. You can see it and an example implementation here.
The library itself is rather simple. There are two methods:
.register(name, fn/prop)
This method will register a function or property that you would like to use as a mixin later for the construction of an object. The name must be unique, else it will overwrite a previously-saved mixin. If you pass null instead of a function or property, then it will unregister that particular named mixin. You can register as many as you would like, and they will be namespaced inside the mkobj library itself.
.construct([name1, name2, name3, …, nameN])
This will return an object with all of the properties passed in the array on it as keys, provided that they were previously registered using .register() (see above). If you didn’t .register() it, then it won’t be set on the object. All objects created by .construct() are instance-safe.
As well, you could take an object created by .construct() and make an instance-safe copy of it using Object.create();.
Have fun, play around with this. Perhaps if I get especially motivated next week before I head back to work, I will do the whole ‘module-compatibility’ thing, so that it’s RequireJS/CommonJS/Bower/Browserify/whatever-compatible. This technique would work well for Node as well. (Which is how I tested it all, anyway).